Python Libraries, Modules & Packages: The Lego Blocks of Code
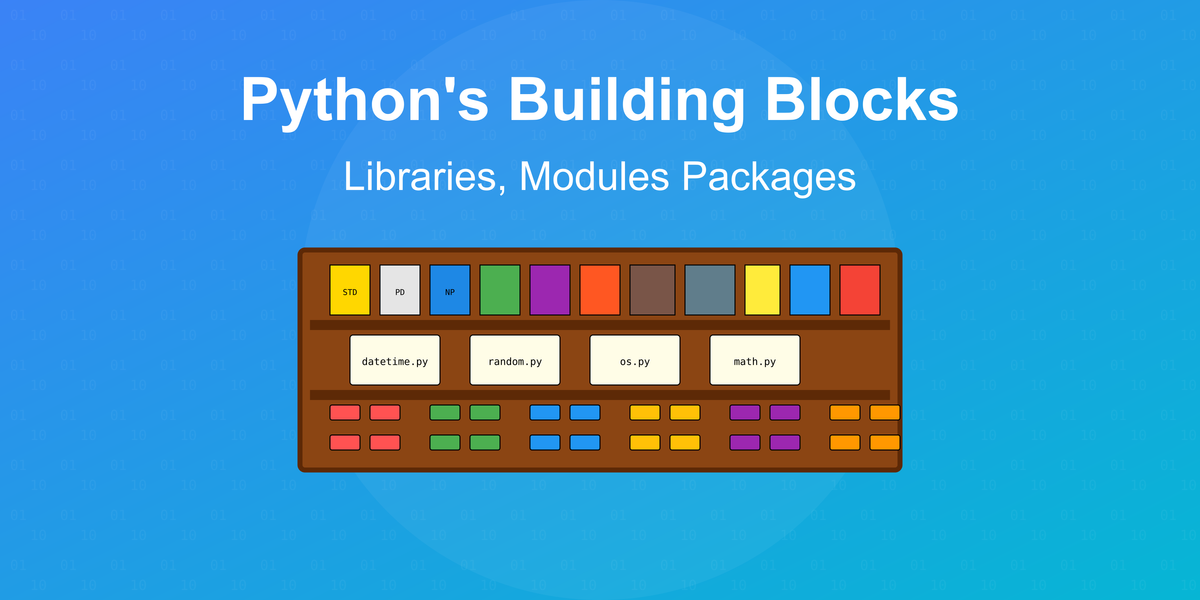
Well libraries, modules and packages in Python are like those essentials in your kitchen pantry that you just can't do without. Think of programming languages as business analysts, they help connect, write, compile, interpret a friendly version of instructional rule-based writing on our machines- and now we are going to explore how to organize these instructions into meaningful, reusable components!
Hope we had a comfortable start!
Alright, lets break down what we call libraries, modules and packages into steps,
- Modules: consider these to be individual recipe cards that your grandmother meticulously wrote down. Each card details a specific dish with its ingredients, quantities, and cooking method. Similarly, a module in Python is a single file (.py) that contains Python definitions, functions, and variables meant for a specific purpose.
- Packages: now imagine your grandmother organized all these recipe cards into different categories- breakfast, lunch, dinner, desserts, and compiled them into a family cookbook with a proper index. That's what a package is! It's a collection of related modules organized in directories with a special
__init__.py
file that tells Python this directory should be treated as a package. - Libraries: envision a massive bookshelf containing cookbooks from various cuisines across the world. You've got Italian, Chinese, Mexican, Indian... That's a library for you! It's a collection of packages designed to be reused across different projects.
Think of it this way- when we say import pandas, it's like saying "I'm planning to cook something that requires techniques from that big data cookbook, so let me bring that cookbook into my kitchen counter space."
Why do we need these organized blocks?
yes they are needed!
to ensure we don't reinvent the wheel, over and over again. For example, If someone has written say a function specific to parsing dates, the moment we have its module equivalent- it can be used in any of your Python projects. There's no need to rewrite parsing logic ever again. We call this the DRY principle: Don't Repeat Yourself!
this is why we call Python the "batteries included" language- it comes with a standard library full of essential tools ready to use!
The Anatomy of a Module
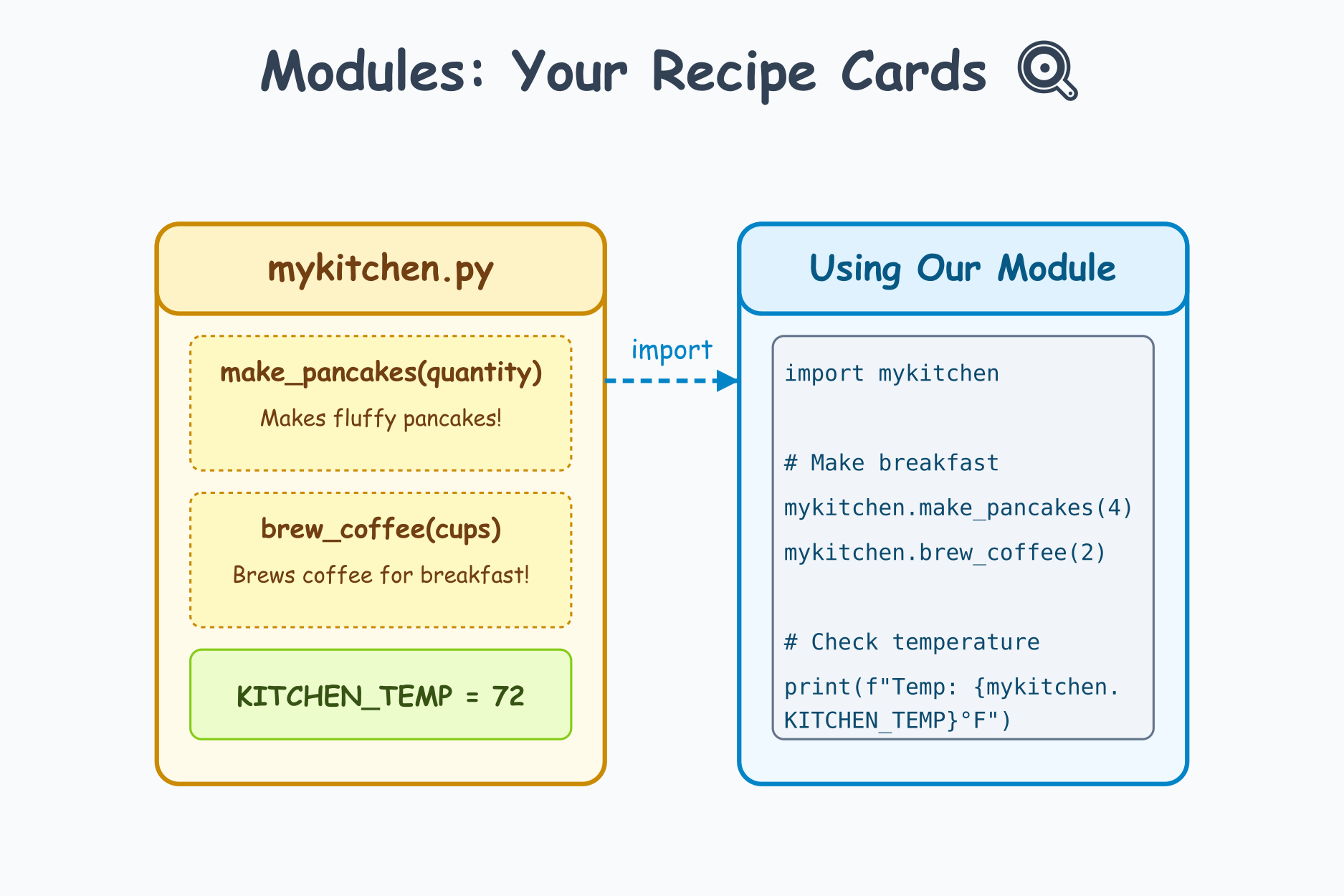
Let's create a simple module ourselves to understand the concept better:
# mykitchen.py
def make_pancakes(quantity):
print(f"Making {quantity} fluffy pancakes!")
return f"{quantity} pancakes ready to serve!"
def brew_coffee(cups):
print(f"Brewing {cups} cups of coffee...")
return f"{cups} cups of coffee ready!"
KITCHEN_TEMP = 72 # A module-level constant
Here, mykitchen.py
is a module with two functions and one constant. To use it:
# Using our module
import mykitchen
# Make breakfast
mykitchen.make_pancakes(4)
mykitchen.brew_coffee(2)
# Check kitchen temperature
print(f"Kitchen temperature is {mykitchen.KITCHEN_TEMP}°F")
Creating a Package
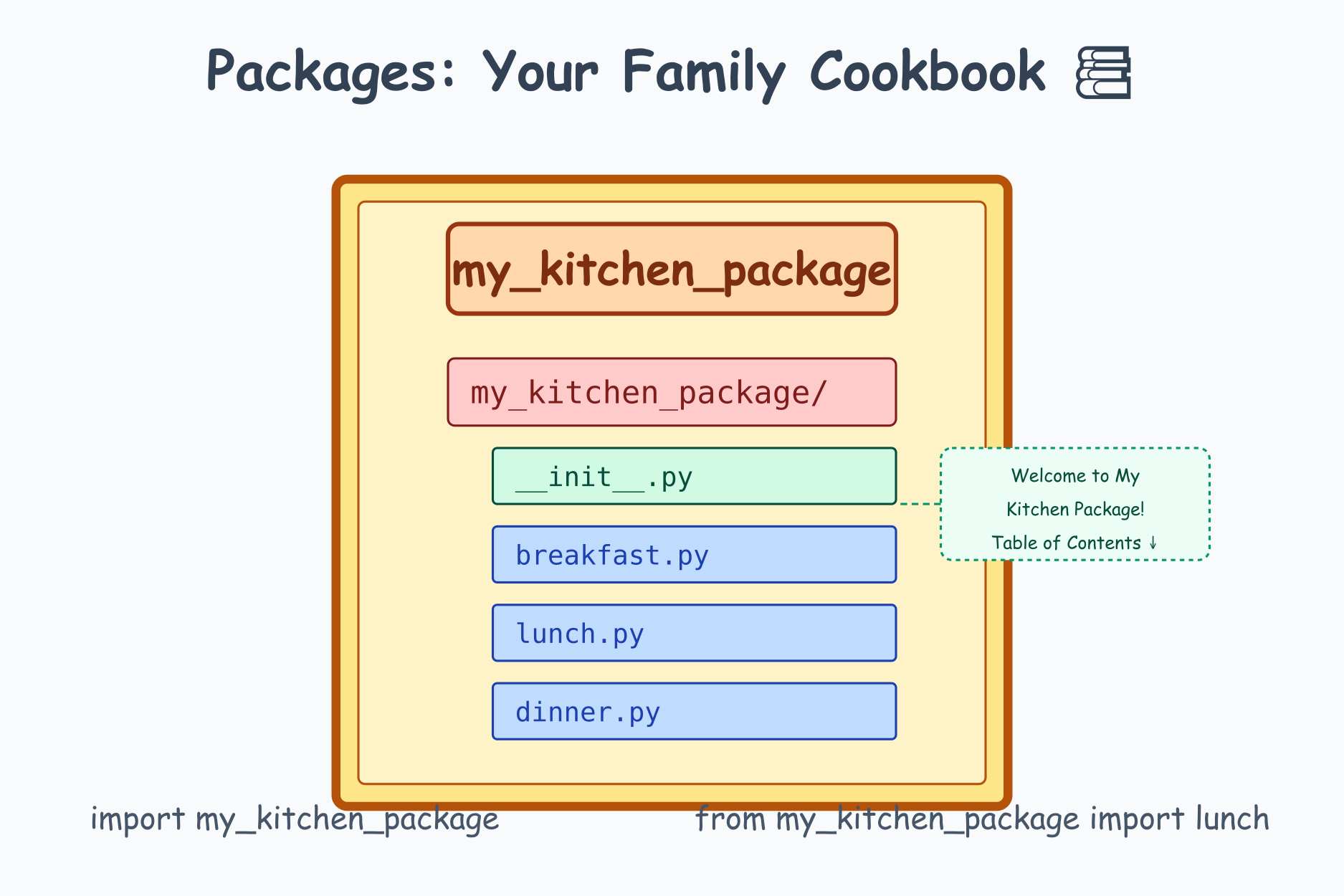
Now, let's organize multiple modules into a package:
my_kitchen_package/
│
├── __init__.py
├── breakfast.py
├── lunch.py
└── dinner.py
The __init__.py
file can be empty, or it can contain initialization code:
# __init__.py
print("Welcome to My Kitchen Package!")
# We can also import specific functions to make them directly accessible
from .breakfast import make_pancakes, brew_coffee
Using our package:
# Import the package
import my_kitchen_package
# Make breakfast using the imported functions
my_kitchen_package.make_pancakes(2) # Works because we imported it in __init__.py
# For functions not imported in __init__.py
from my_kitchen_package import lunch
lunch.make_sandwich("ham and cheese")
The Standard Library: Python's Built-in Cookbook
Python comes with a rich standard library that's like a set of essential cookbooks every Python chef gets for free:
# Using datetime module from the standard library
import datetime
# What's today's date?
today = datetime.date.today()
print(f"Today is {today}")
# Using random module
import random
# Let's randomly select a meal
meals = ["pasta", "salad", "soup", "steak"]
dinner = random.choice(meals)
print(f"Tonight's dinner: {dinner}")
Finding the Right Tool: External Libraries
While Python's standard library is comprehensive, sometimes you need specialized tools. That's where external libraries come in:
# Using pandas for data analysis
import pandas as pd
# Read data from CSV file
data = pd.read_csv("sales_data.csv")
# Quick analysis
total_sales = data["amount"].sum()
print(f"Total sales: ${total_sales}")
Before you can use external libraries like pandas, you need to install them:
pip install pandas
Virtual Environments: Separate Kitchens for Different Experiments
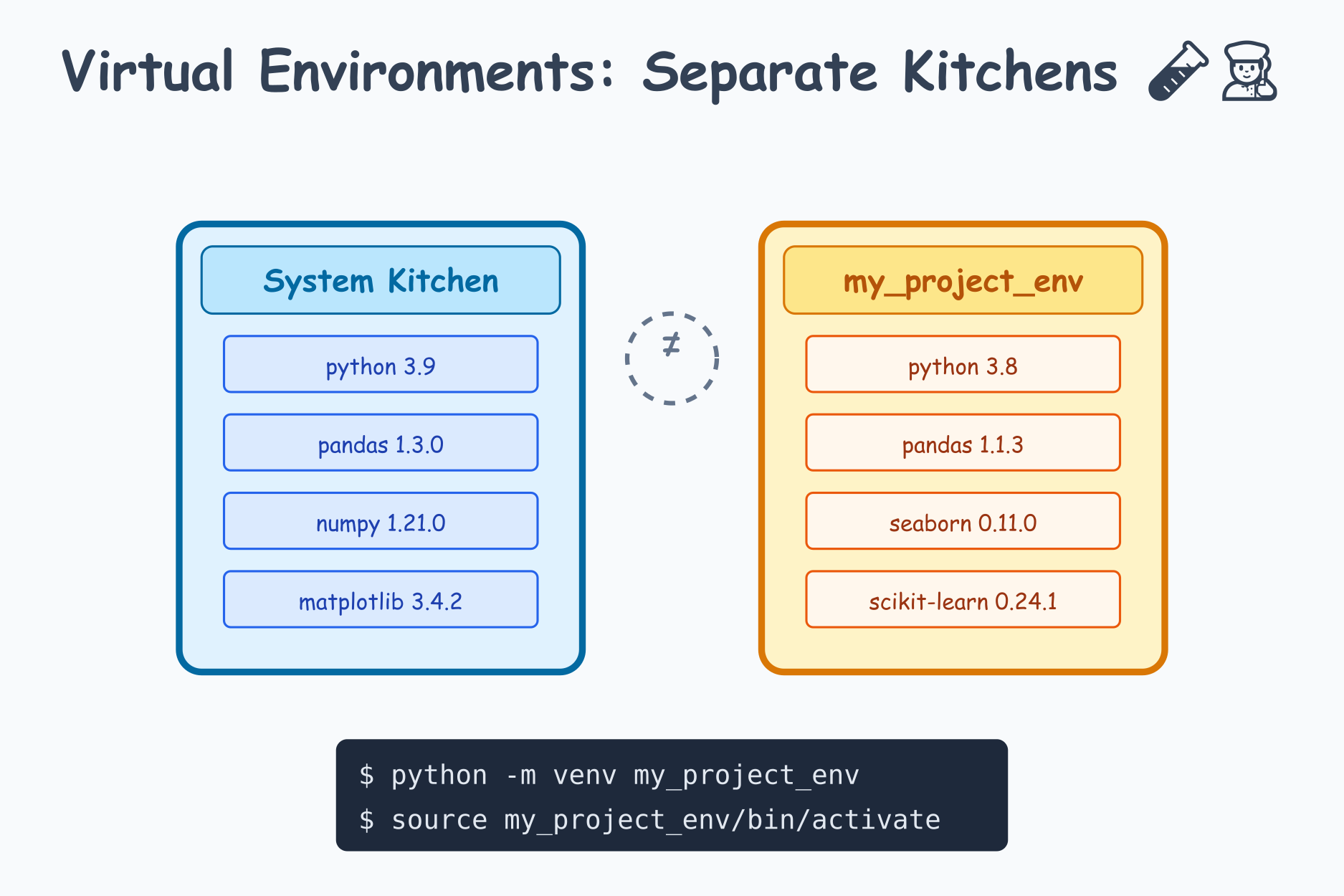
okk take a breather,
Imagine you're testing two recipes that require different versions of the same ingredient. You wouldn't want them to interfere with each other, right? Virtual environments serve the same purpose:
# Create a virtual environment
python -m venv my_project_env
# Activate it (Windows)
my_project_env\Scripts\activate
# Activate it (macOS/Linux)
source my_project_env/bin/activate
# Install packages in this isolated environment
pip install pandas matplotlib seaborn
# When done, deactivate
deactivate
Creating Your Own Reusable Kitchen Tools
As you become more experienced, you'll create functions and classes that you want to reuse across projects. This is where creating your own modules and packages becomes invaluable.
Let's say you've created some amazing data cleaning functions. Package them up!
data_cleaner/
│
├── __init__.py
├── text_cleaner.py
├── numeric_cleaner.py
└── date_cleaner.py
And now you can distribute your package or just copy it between projects.
Best Practices for Working with Modules and Packages
- Import only what you need:
from math import sqrt
is better thanimport math
if you only need the square root function. - Use standard aliases:
import pandas as pd
andimport numpy as np
are conventional and make your code more readable to others. - Keep your modules focused: Each module should have a clear, single responsibility.
- Document your modules: Use docstrings to explain what your modules, functions, and classes do.
- Manage dependencies: Use requirements.txt or setup.py to track and specify your project's dependencies.
Why does this matter to a data practitioner?
For data engineers and data scientists, mastering the use of libraries, modules, and packages is not just convenient—it's essential. Most data work in Python relies heavily on specialized libraries like pandas, numpy, matplotlib, scikit-learn, and others.
Furthermore, organizing your own code into well-structured modules and packages makes it:
- More maintainable (easier to update)
- More reusable (no copying and pasting between projects)
- More shareable (easier for colleagues to understand and use)
- More testable (easier to write unit tests)
Think of it this way: good chefs don't just know how to follow recipes—they know how to organize their kitchen, where to find the right tools, and how to efficiently move between different cooking tasks. Similarly, good Python data practitioners don't just write code—they organize it into logical, reusable components.
What's Next?
We will uncover a plethora of advanced packaging concepts, virtual environments and dependency management at a deeper level! Just stick around, and definitely subscribe if you haven't yet..
Stay curious, keep coding, and happy kitchening... I mean, Pythoning! Cheers!