Python: Errors & Exceptions - When Your Code Has a Meltdown
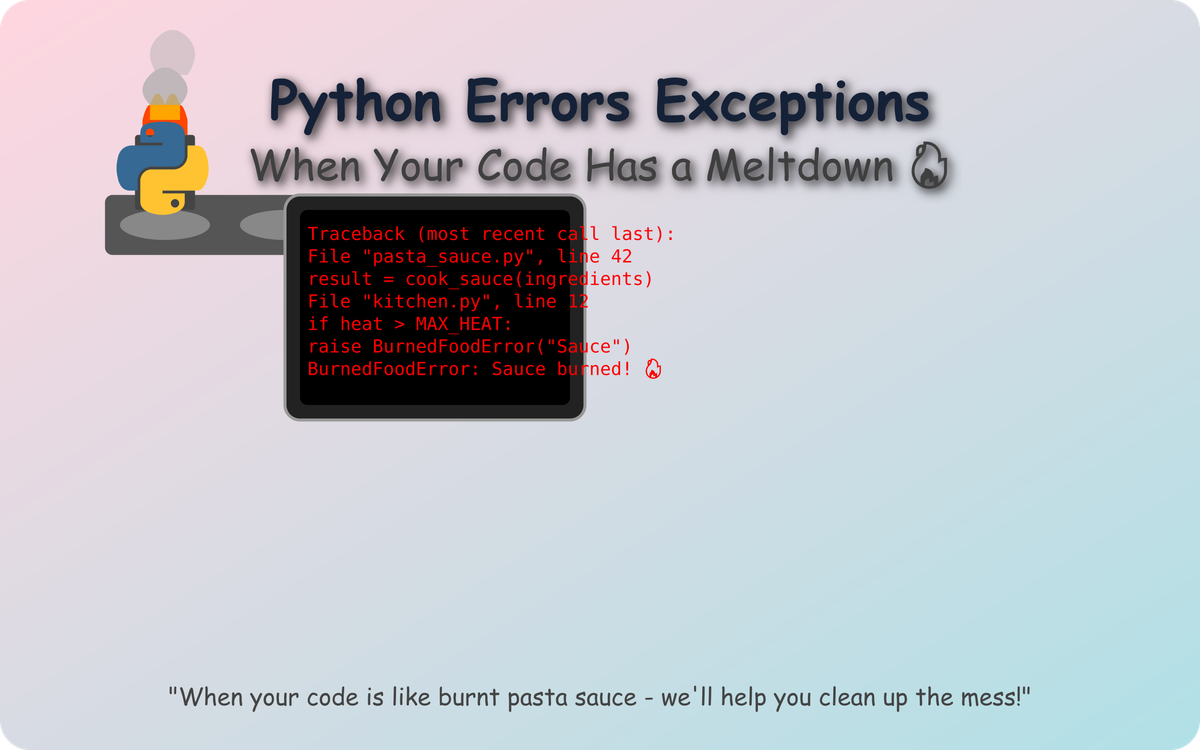
Last weekend I was trying to make my grandma's special pasta sauce - you know, the one with the secret ingredient that she'd never tell anyone about (I think it's just extra butter, honestly). Anyway, I got distracted by a YouTube video about capybaras taking baths, and my sauce burned BADLY. Like, "setting off every smoke detector" badly.
And you know what? That's EXACTLY what Python errors feel like. One minute you're confidently writing code, the next minute EVERYTHING IS ON FIRE and your terminal is screaming at you with red text.
Hope we had a comfortable start! Let's dive into this error-filled swimming pool together.
The "Oops" Moments in Python
When Python runs into problems, it doesn't just quietly fail like my first relationship. Oh no. It TELLS you about it, sometimes in excruciating detail. This is actually a good thing! It's like when your friend tells you there's spinach in your teeth - embarrassing, but better than walking around all day like that.
There are three main types of "oops moments" in Python:
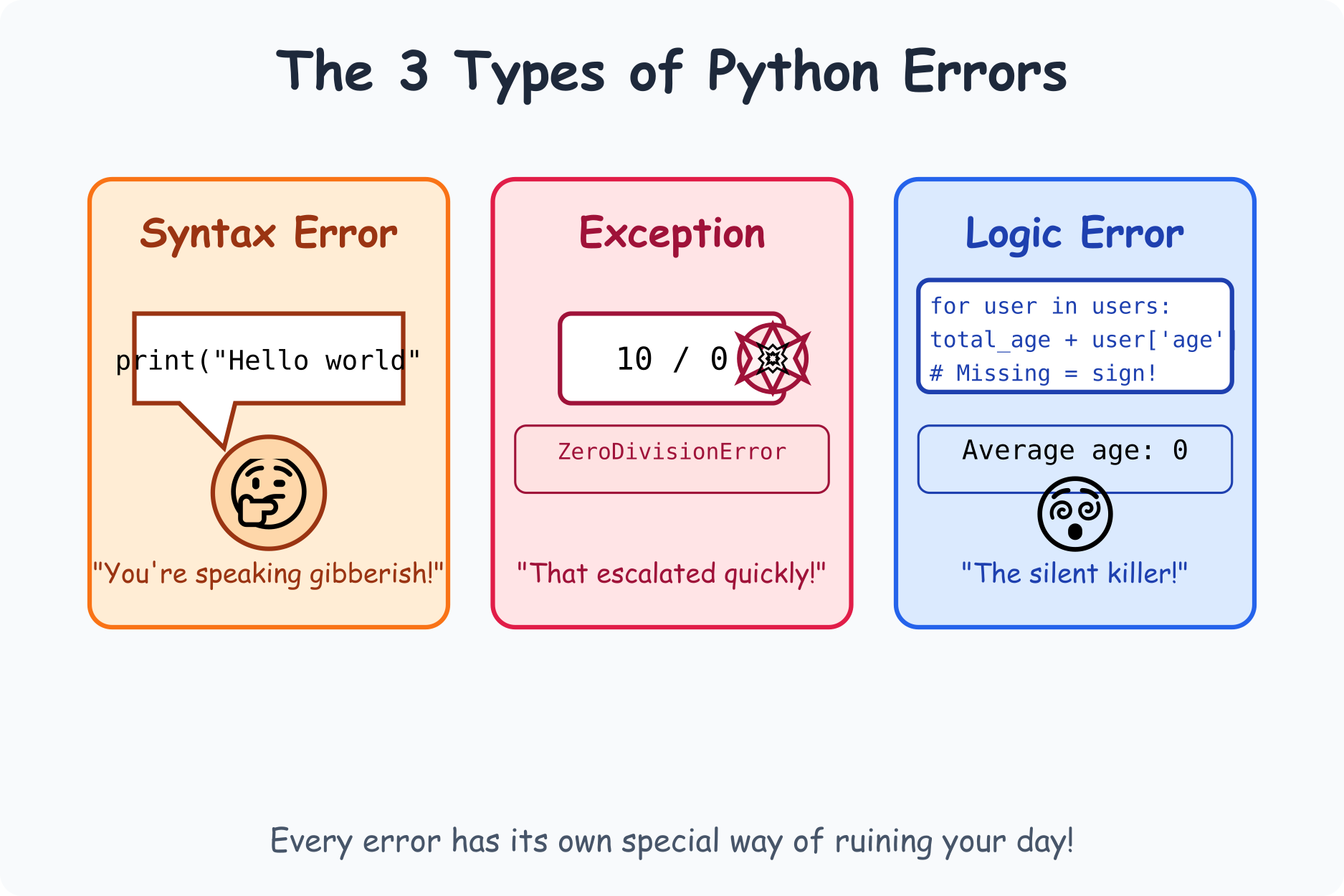
Syntax Errors: The "You're Speaking Gibberish" Problem
Think of syntax errors as trying to order food in a foreign language using Google Translate and accidentally asking for a "fried telephone with extra clouds."
# This is me trying to print hello but forgetting to close the parenthesis
print("Hello world"
Python's like: "What? I don't understand what you're trying to say. Are you done? Hello???"
I sometimes stare at these errors for like 20 minutes before realizing I forgot a stupid colon or something. We've all been there!
Exceptions: The "Well, That Escalated Quickly" Problem
Your code LOOKS correct, Python understands what you're TRYING to do, but then something goes horribly wrong when you actually run it.
Like that time I tried to divide by zero in 3rd grade and my math teacher had an existential crisis:
# Me trying to be clever
result = 10 / 0 # Boom! ZeroDivisionError
Or that time I tried to access data that wasn't there:
my_list = [1, 2, 3]
print(my_list[99]) # IndexError - there's no 100th element, silly!
Logic Errors: The Silent Killers
These are the WORST. Your code runs perfectly. No errors. But the results are completely wrong because YOUR logic was wrong.
It's like following a cake recipe perfectly but still ending up with a brick because the recipe itself was bad. I once spent THREE DAYS hunting down a logic error that turned out to be me using +
instead of *
in one line of code. THREE. DAYS.
# Calculating average age of users
total_age = 0
num_users = len(users)
for user in users:
total_age + user['age'] # Oops! I'm not actually adding anything to total_age!
average_age = total_age / num_users # This will always be 0!
That little mistake cost my company approximately 30 cups of stress-coffee.
Catching Exceptions: Like Installing Safety Nets for Your Code
In real life, we anticipate problems. We carry umbrellas when it might rain. We have insurance for our cars. We have a backup plan when our favorite takeout place is closed.
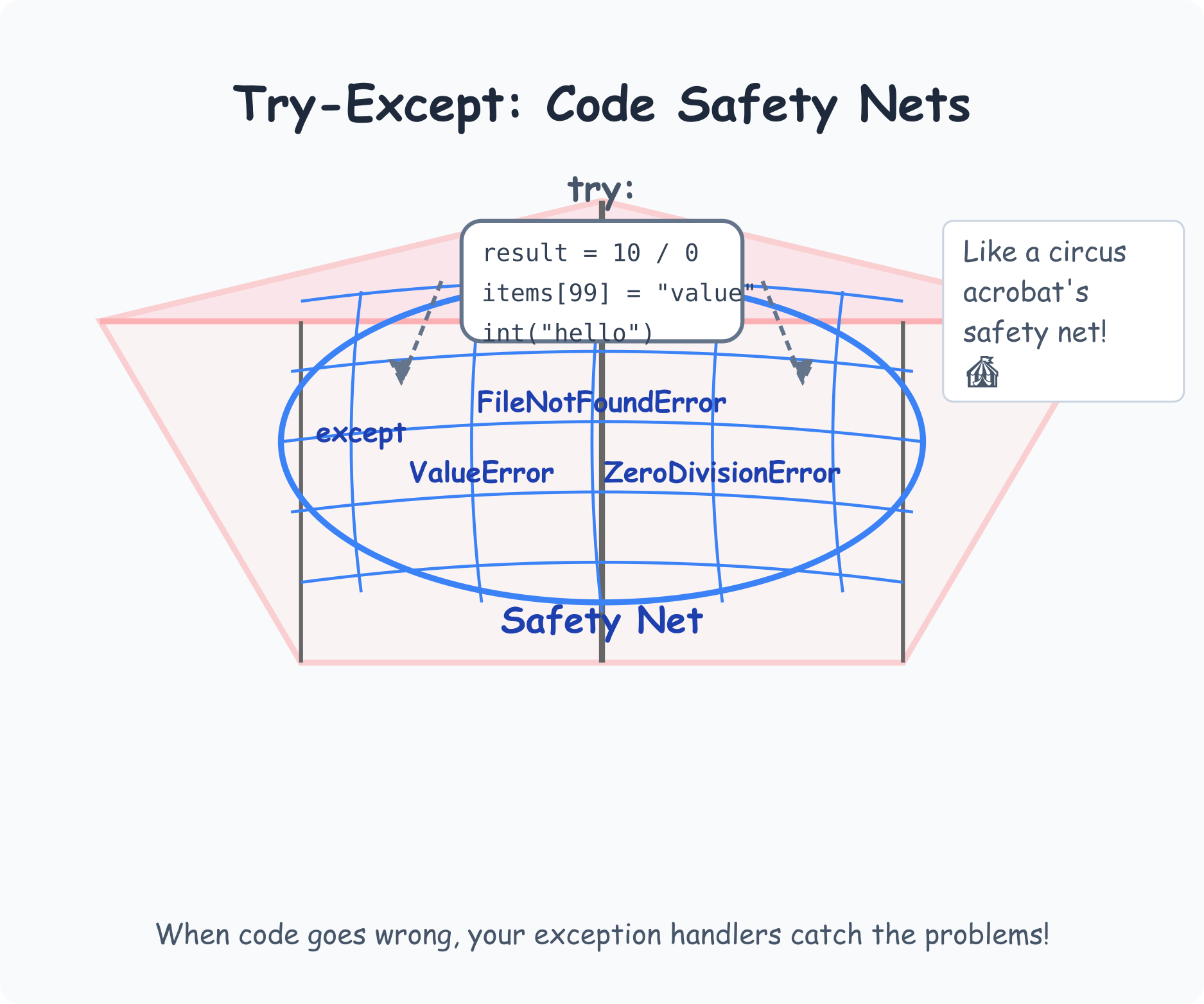
In Python, we use try-except
for this:
# Me trying to convert user input to a number
user_input = input("Enter a number: ")
try:
number = int(user_input)
result = 100 / number
print(f"100 divided by your number is: {result}")
except ValueError:
print("That's not a number! Did you even graduate elementary school?")
except ZeroDivisionError:
print("Dividing by zero? Are you TRYING to break mathematics?")
okk take a breather,
The structure works like this:
- The
try
block is your optimistic code. "This is TOTALLY gonna work!" - The
except
blocks are your backup plans. "But juuuust in case..."
The 'Ugh, Fine, I'll Clean Up' Phase: finally
Sometimes you need to do something regardless of whether there was an error or not. Like cleaning the kitchen after cooking, whether dinner was a success or a disaster.
file = None
try:
file = open("important_data.txt", "r")
content = file.read()
print(f"File says: {content}")
except FileNotFoundError:
print("Uh oh, file is missing! Did the dog eat your homework again?")
finally:
if file:
file.close() # Always close your files, kids!
print("File closed. I'm a responsible adult!")
Making Up Your Own Error Types: When Being Dramatic is Justified
Sometimes the built-in exceptions just don't capture the SPECIFIC way things went wrong. That's when you can create your own exception classes:
class IncrediblyLowBatteryError(Exception):
"""Raised when your laptop battery is about to DIE DIE DIE."""
pass
def check_battery(percentage):
if percentage < 5:
raise IncrediblyLowBatteryError(f"ONLY {percentage}% BATTERY LEFT! PANIC MODE ACTIVATED!")
elif percentage < 20:
print("You should probably find a charger soon...")
else:
print("Battery level is fine, keep scrolling Twitter.")
try:
check_battery(3)
except IncrediblyLowBatteryError as e:
print(f"Emergency! {e}")
print("Saving all work and shutting down...")
Real-Life Examples That Actually Happened to Me
1. The Missing CSV Nightmare
I once wrote a data pipeline that processed files from a specific directory. It worked PERFECTLY in testing. Then we deployed it to production and...
try:
df = pd.read_csv("customer_data_q2.csv")
# Process the data...
except FileNotFoundError:
# This actually happened to me! The file naming convention
# changed from customer_data_q2.csv to Q2_customer_data.csv
print("File not found! Checking for alternative naming patterns...")
for file in os.listdir('./data'):
if 'customer' in file and 'q2' in file.lower():
print(f"Found possible match: {file}")
df = pd.read_csv(f"./data/{file}")
break
This saved me from a 2 AM emergency call!
2. The API Timeout Drama
Working with external APIs is like dealing with that one flaky friend who might or might not show up to your birthday party:
import requests
import time
def get_data_with_retry(url, max_retries=3):
retries = 0
backoff = 2 # seconds
while retries < max_retries:
try:
response = requests.get(url, timeout=10)
response.raise_for_status() # Raise exception for 4XX/5XX status codes
return response.json()
except (requests.exceptions.Timeout,
requests.exceptions.ConnectionError):
retries += 1
if retries < max_retries:
print(f"Connection failed! Retrying in {backoff} seconds...")
time.sleep(backoff)
backoff *= 2 # Exponential backoff
else:
print("I give up! This API hates me!")
raise
except requests.exceptions.HTTPError as e:
if response.status_code == 429: # Too Many Requests
print("Server says we're being too needy. Backing off...")
time.sleep(30)
retries += 1
else:
print(f"HTTP Error: {e}")
raise
The Art of Reading Error Messages
Error messages are like those weird medical symptoms you Google at 3 AM - scary at first, but actually helpful if you know how to interpret them.
Here's my approach:
- Start from the bottom - the actual error is usually the last line
- Work your way up - trace how you got there
- Look for line numbers - they tell you exactly where to check
- Ignore the scary-looking parts - those long paths to library files usually aren't your problem
My Personal Rules for Exception Handling
After years of making mistakes (SO MANY MISTAKES), here's what I've learned:
- Don't catch exceptions you don't understand - it's like catching a wild animal without knowing if it's a kitten or a tiger
- Be specific - catching
Exception
is like using a shotgun to kill a fly - Log everything - your future self will thank you when debugging at midnight
- Never do this:
try:
# Some code that might fail
except Exception:
pass # The "I don't care what happened" approach
This is like putting a piece of tape over your car's check engine light. The problem doesn't go away, you just can't see it anymore!
When Debugging Gets REALLY Frustrating
I once spent an entire day debugging a function that kept giving me weird results. I was getting desperate and added print statements EVERYWHERE:
def process_data(data):
print("Starting to process data")
print(f"Data received: {data}")
result = 0
print("Initialized result to zero")
for i, value in enumerate(data):
print(f"Processing item {i}: {value}")
temp = value * 2
print(f"Doubled to: {temp}")
result += temp
print(f"Current result: {result}")
print(f"Final result: {result}")
return result
My terminal looked like a teenager's text message history. But guess what? It WORKED. I found the bug!
When things get REALLY bad, there's always the nuclear option - the Python debugger:
import pdb
def mysterious_function(data):
result = []
for item in data:
# Something's going wrong around here...
pdb.set_trace() # This drops you into interactive debugging mode
processed = complex_calculation(item)
result.append(processed)
return result
In Conclusion: Embrace the Errors!
Errors aren't your enemy - they're like those brutally honest friends who tell you when your new haircut looks terrible. It hurts, but it's good for you in the long run!
The difference between junior and senior programmers isn't that seniors make fewer mistakes - we ALL make mistakes. The difference is that senior programmers:
- Know how to interpret errors faster
- Have better strategies for finding and fixing bugs
- Build safety nets into their code from the start
In our next letter, we'll explore Python's testing frameworks - because the only thing better than handling errors gracefully is preventing them entirely!
Just stick around, and definitely subscribe if you haven't yet..
Till then, may your exceptions be caught and your logic be sound. And remember - even code written by NASA has bugs, so don't be too hard on yourself when your function implodes! Cheers!