Python: and we function with functions
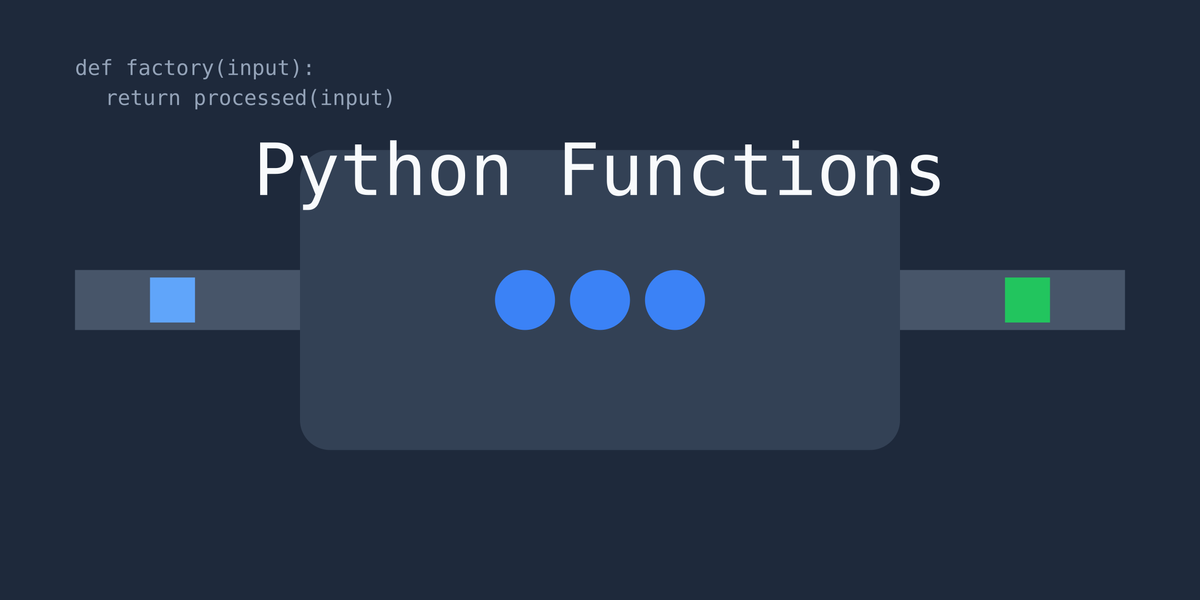
what is a function?
it is a block of code, that is reusable. Of course serves a common purpose when it comes to the outcome or output expected. Just like machineries inside a factory, that are fed some resources or raw materials, with the expectation of a more refined product.
why is it necessary?
alright, let's take up a practical example. say you need to settle a few set of expenses every month, and there are lets assume only two modes of transfers available- one is writing a cheque, and the other is logging into your internet banking, and initiating the same from there.
which would you rather do? netbanking, right? why? since, the process of sending money to your beneficiary, over the internet is similar to writing a cheque, but every time you'll not be required to pull out a cheque leaf for the same- rather pass/submit some standardised set of info, like the receivers name, and bank details. come to think of it you are just replacing the action of pulling out a cheque leaf, with a reusable process or function which expects similar set of data and moves ahead with its process.
this is also referred to as DRY principle that states Don't Repeat Yourself!
Let's dive into a few examples:
def greetUser(name): #function definition with parameter
"""This is a docstring - it explains what the function does"""
greeting = f"Hello, {name}" #Processing
return greeting #return value
The above is a basic structure but that doesn't mean there's anything complex about functions- don't worry
Now lets break it down to its individual constituent components:
- the name: function name i.e. greetUser. Prefer having something that alludes to the purpose the function serves
- parameters: herein we have one i.e. name. This is an information that your function is expected to work on or with. Its a key ingredient that your function expects to receive
- function body: where we mention the steps, and these are your steps that can be referred to often to get things done. repeatable, so that you don't write it multiple times across the code
- a return value: can only be one. function has the ability to return a values, similar to a semi-finished or finished product from factory machinery
Now, broadly we can look to classify function into certain types. It not that they have different names in particular, but it all depends in the nature of use case it is supposed to work on.
- Has No parameters and No return value
def sayHello():
print("Hello!")
- Has parameters and No return value
def printSum(a, b):
print(f"Sum is: {a+b}")
- Has parameters and Returns value
def calculateSum(a, b):
return a + b
- Has parameters With default value
def greet(name = "User"):
return f"Hello, {name}!"
Now, lets look at Function Scope.
Think of functions to have their own shelf atop the kitchen counter space that they create for themselves in memory- thereby giving rise to global and local scope. Here's an example:
x = 10 # global variable
def modifyNumber():
x = 20 # local variable, not the same as x we have outside this function scope
print(f"Inside function: {x}")
modifyNumber() # calling the function, prints 20
print(f"Outside function: {x}") # and this one will give us 10
Before we wrap, lets also look at a few advanced features that Python function provides us, when it comes to unwrapping and accessing an unknown number of parameters passed to a function during its call.
def sumAll(*args): # we expect any number of arguments here
return sum(args)
def userInfo(**kwargs): # arguments that are ready to accept keyword / key value paired
for key, value in kwargs.items():
print(f"{key}: {value}")
square = lambda x: x**2 # lambda functions i.e. small anonymous functions
lambda? it's simple. Think of them as an enabler where in were are able to strip of those ceremonious traditional function syntax, simple to read, where on one side you have an input, on the other a simple straight outcome. Finally, they are created without an intent to persist, created for use just in the moment.
We will look into more of such, as we move ahead in our journey. Hope this was a fun read, see you around!